Summary: in this tutorial, you will learn how to use the Perl last statement to exit a loop immediately.
Introduction to the Perl last statement
The Perl last
statement is used inside a loop to exit the loop immediately. The last statement is like the break
statement in other languages such as C/C++, and Java.
In practice, you often use the last
statement to exit a loop if a condition is satisfied e.g., you find an array element that matches a search key, therefore, it is not necessary to examine other elements.
Because of this, you typically use the last
statement in conjunction with a conditional statement such as if or unless .
The following illustrates how to use the last
statement inside a while or for loop statement.
Inside a while
statement (the same for the until statement):
while(condition){
# process something
last if(expression);
# some more code
}
Code language: Perl (perl)
Inside a for
loop statement:
for(@a){
# process current element
last if(condition);
# some more code
}
Code language: Perl (perl)
For the do while and do until statement, you have to use an additional block {}
as described in more detail in the corresponding tutorial.
The following flowchart illustrates how the last
statement works inside a while
loop statement.
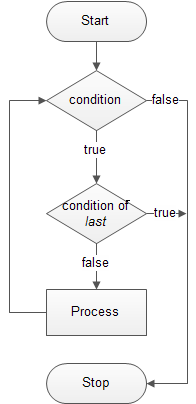
Perl last statement examples
Let’s start with a simple example to find an element in a hash by a given key.
#!/usr/bin/perl
use warnings;
use strict;
my ($key, $value);
my %h = ("apple" => 1,
"orange" => 2,
"mango" => 3,
"coconut" => 4);
print("Please enter a key to search:\n");
$key = <STDIN>;
chomp($key);
$value = 0;
# searching
foreach(keys %h){
if($_ eq $key){
$value = $h{$_};
last; # stop searching if found
}
}
# print the result
if($value > 0){
print("element $key found with value: $value\n");
}else{
print("element $key not found\n");
}
Code language: Perl (perl)
How program works.
- First, we looped over the elements of the hash and compared each hash key with the search key.
- Second, inside the loop, if we found the match, we exited the loop immediately by using the
last
statement without examining other elements. - Third, we displayed the result of the search.
Perl last statement with loop label example
If you use the last
command alone, it exits only the innermost loop. In case, you have a nested loop that is a loop, called inner loop, nested inside another loop, called outer loop. If you want to exit both the inner and outer loop you need to:
- Put a label in the outer loop.
- Pass the label to the
last
statement.
The following example demonstrates how to use the last
statement with a label:
#!/usr/bin/perl
use warnings;
use strict;
my ($key, $value);
my %h = ("apple" => 1,
"orange" => 2,
"mango" => 3,
"coconut" => 4);
$value = 0;
print("Please enter a key to search:\n");
OUTER: while(<STDIN>){
# get input form user
$key = $_;
chomp($key);
# seaching
INNER: foreach(keys %h){
if($_ eq $key){
$value = $h{$_};
last OUTER; # exit the while loop
}
} # end for
print("Not found, Please try again:\n") if($value == 0);
}# end while
print("element found with value: $value\n");
Code language: Perl (perl)
In this tutorial, we have shown you how to exit the loops immediately by using the Perl last
statement.